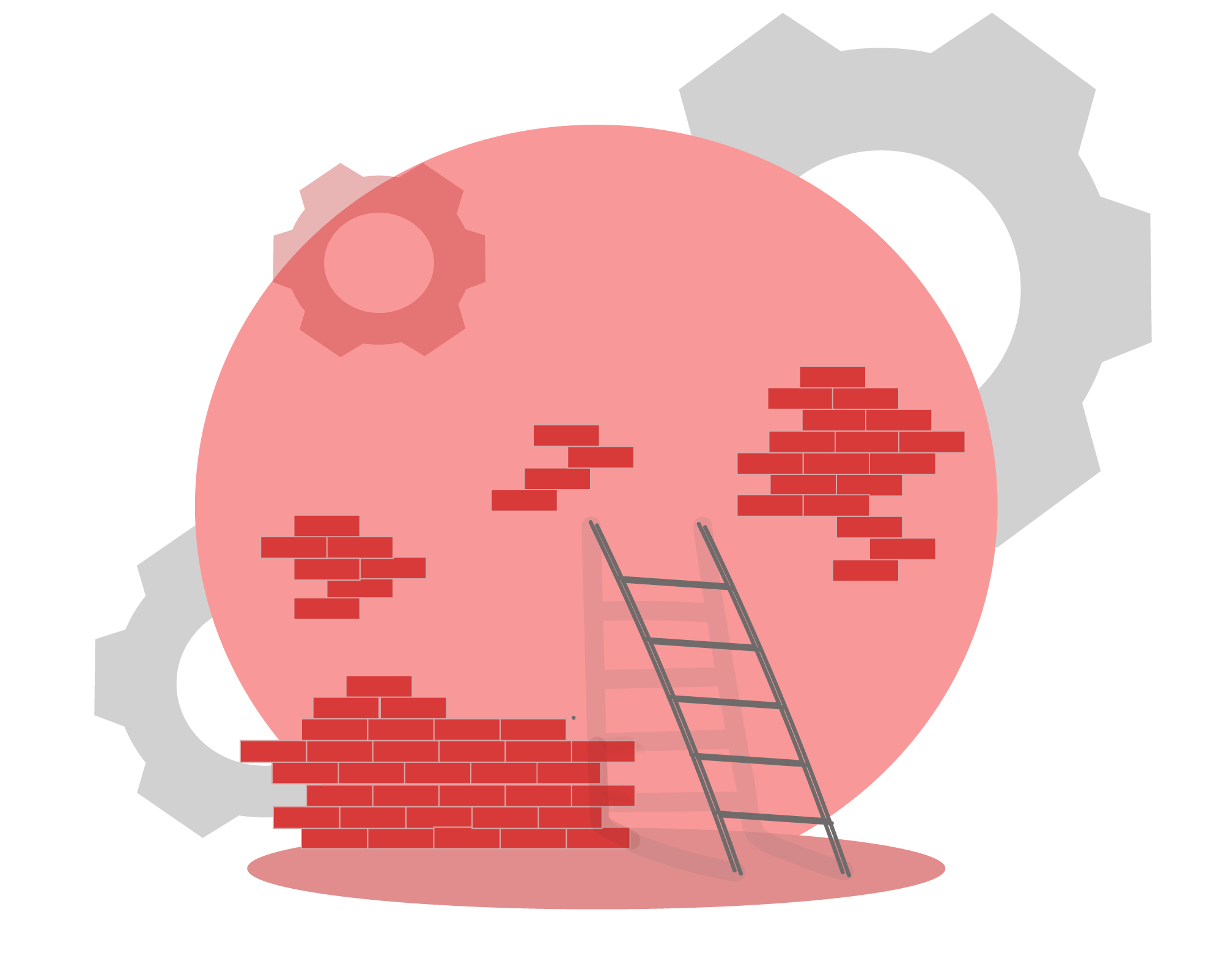
Advanced Builder Using Java 8 Lambda
Disclaimer : The content of this blog are my views and understanding of the topic. I do not intend to demean anything or anyone. I am only trying to share my views on the topic so that you will get a different thought process and angle to look at this topic.
Introduction
Java 8 introduced Functional Programming using Lambdas which enables us to externalize the behaviour (i.e. a function) that can be passed as an argument to another function. Looks simple, but it’s a very powerful feature! I was fascinated by this idea and wanted to experiment with it. My experimentation is always based on what language restrictions are and how far can I reach based on my skills, knowledge and creativity. I created something called an Advanced Builder using this. Let’s explore how.
We all know that a Builder solves the problem of a telescopic constructor while instantiating an object. In case of a constructor with many arguments, it’s difficult to read quickly which argument is to be passed in which position. IDE tools nowadays are smart enough to show in the code the parameter names, but still, there are certain cases when they don’t show them. My observation is that they show the parameter names as far as we pass actual values as arguments and not a method call as direct argument.
Please see the screenshot below :

How to solve this problem using Java 8 Lambda and create a builder using the same?
Check the code below and let’s discuss more on this:
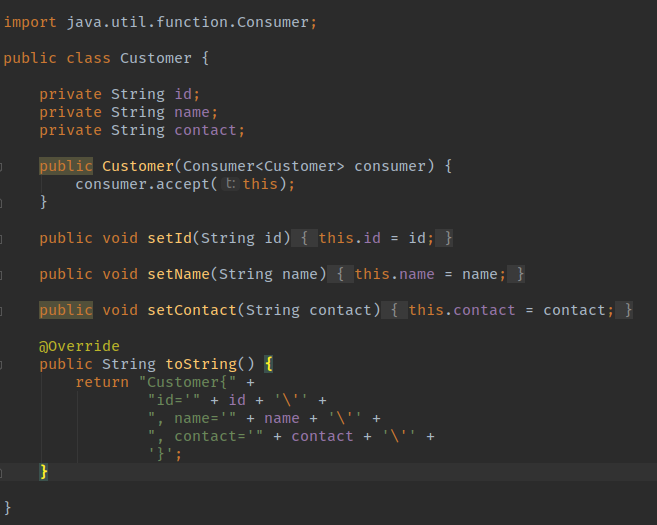

Below are some points to be noted on this:
PROS
- Object can be constructed in various ways
- Object building logic is externalized using a Consumer Lambda
- The code is readable when we are building an Object as we know which particular setter method is invoked
- Single constructor for all the different ways of object creation
CONS
- Cannot create Immutable objects using this approach
I recall a friend of mine once wrote a beautiful blog on Advanced Builder Pattern using Lambda. In his approach he used an externalized Builder and I am using the same approach in a different way. I used an Inner Builder Class to achieve the Immutability.


Structural Analysis
Structural analysis of the above class reveals:
- Both Customer and Bulider class have private constructor, which means we cannot instantiate them directly
- We can create only Immutable objects of Customer with the help of the Inner Builder Class
- The with method in the builder class accepts a Consumer which is telling the builder how to build the objects of Customer
- build method inside the Builder class is private which means we cannot invoke it directly and Builder has clearly defined the invocation order in the with method
- The lifespan of the builder object is limited to instantion of Customer based on the states defined by Consumer
In simple words, we are telling the builder what the state of the Customer instance which we need to build it with are. It takes that, applies it, generates an instance of Customer, and returns it back. Now we can create Immutable Objects of Customer with much more readable code.
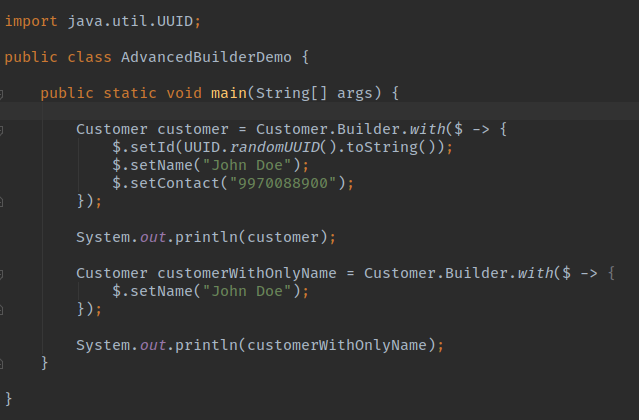

Summary
Java 8 Lambdas are an addition to the freedom provided to Developers by Java. We can create smarter code and make it more Readable, Reusable and AGILE. I tried experimenting so far to create this Advanced Builder but I am trying to achieve how we can get rid of this Inner Builder class, have the single constructor in the original class, and create Immutable Objects.